Hosted checkout
xMoney offers a secure, hosted checkout experience. This allows your customers to easily select their preferred payment method and complete their purchase. Our hosted checkout is designed for optimal performance and conversion, ensuring a smooth transaction.
Checkout initialization
To initiate the xMoney checkout and redirect your customer for payment, you need to create an HTML form with two essential parameters: jsonRequest
and checksum
.
jsonRequest
: A JSON object containing all transaction details, base64 encoded.checksum
: An alphanumeric sequence (base64 encoded) that verifies the JSON's integrity, preventing tampering.
Here's an example of a JSON object containing transaction details:
{
"siteId": 1,
"customer": {
"identifier": "your-unique-customer-id",
"firstName": "John",
"lastName": "Doe",
"country": "RO",
"city": "Bucharest",
"email": "john.doe@test.com"
},
"order": {
"orderId": "your-unique-order-id",
"description": "Order Description",
"type": "purchase",
"amount": 2194.98,
"currency": "RON"
},
"cardTransactionMode": "authAndCapture",
"backUrl": "https://myshop.com/payment-back-url"
}
To calculate the checksum
and jsonRequest
, you will need to base64 encode the json, and calculate the hmac using your Private Key.
Here are some examples of how to calculate the base64 encoded json and checksum.
const crypto = require('crypto');
function getBase64JsonRequest(orderData) {
const jsonText = JSON.stringify(orderData);
return Buffer.from(jsonText).toString('base64');
}
function getBase64Checksum(orderData, secretKey) {
const hmacSha512 = crypto.createHmac('sha512', secretKey);
hmacSha512.update(JSON.stringify(orderData));
return hmacSha512.digest('base64');
}
// Sample order data
const orderData = {
"siteId": 1,
"customer": {
"identifier": "your-unique-customer-id",
"firstName": "John",
"lastName": "Doe",
"country": "RO",
"city": "Bucharest",
"email": "john.doe@test.com"
},
"order": {
"orderId": "your-unique-order-id",
"description": "Order Description",
"type": "purchase",
"amount": 2194.98,
"currency": "RON"
},
"cardTransactionMode": "authAndCapture",
"backUrl": "https://myshop.com/payment-back-url"
};
const secretKey = "your-private-key";
const base64JsonRequest = getBase64JsonRequest(orderData);
const base64Checksum = getBase64Checksum(orderData, secretKey);
Once you have the base64JsonRequest
and base64Checksum
, generate an HTML form. You have two options for how the customer will be redirected to the xMoney checkout page:
Upon redirection, the customer will be presented with the xMoney hosted checkout page, as illustrated below:
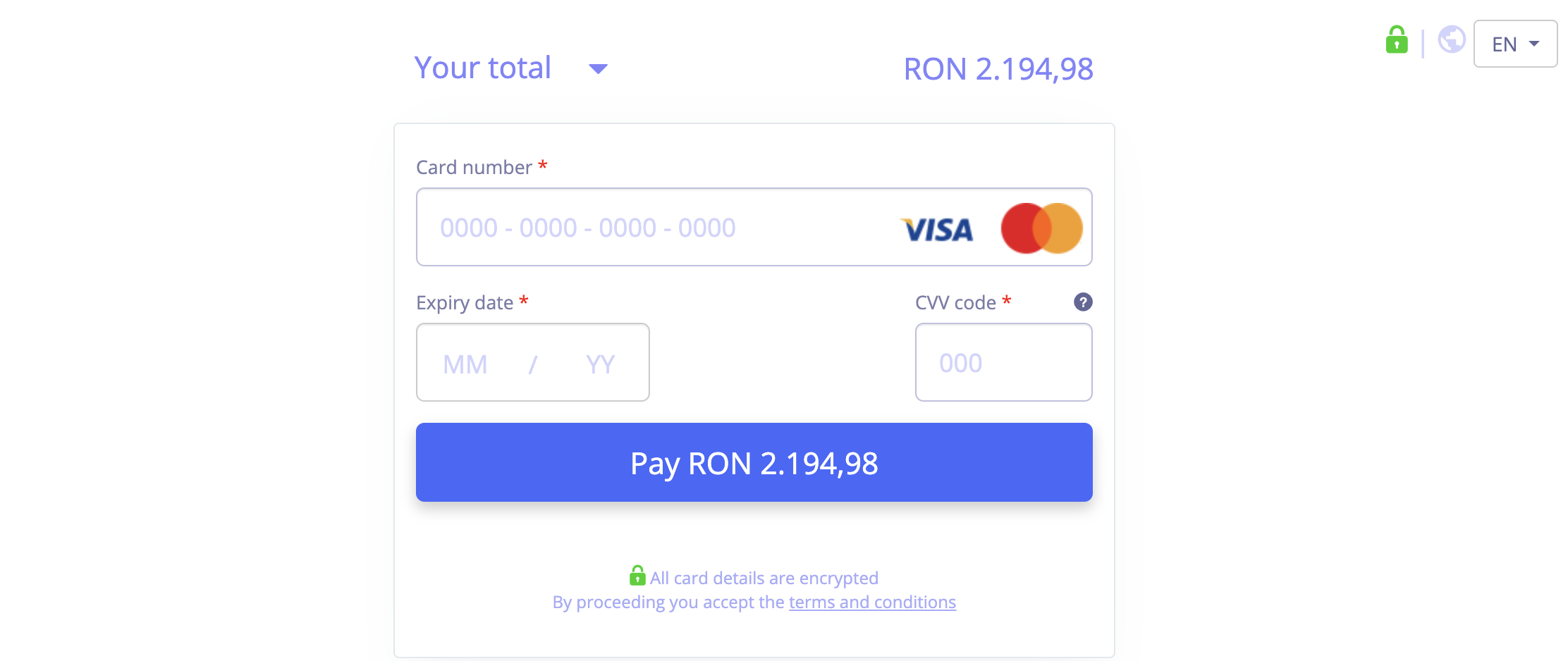
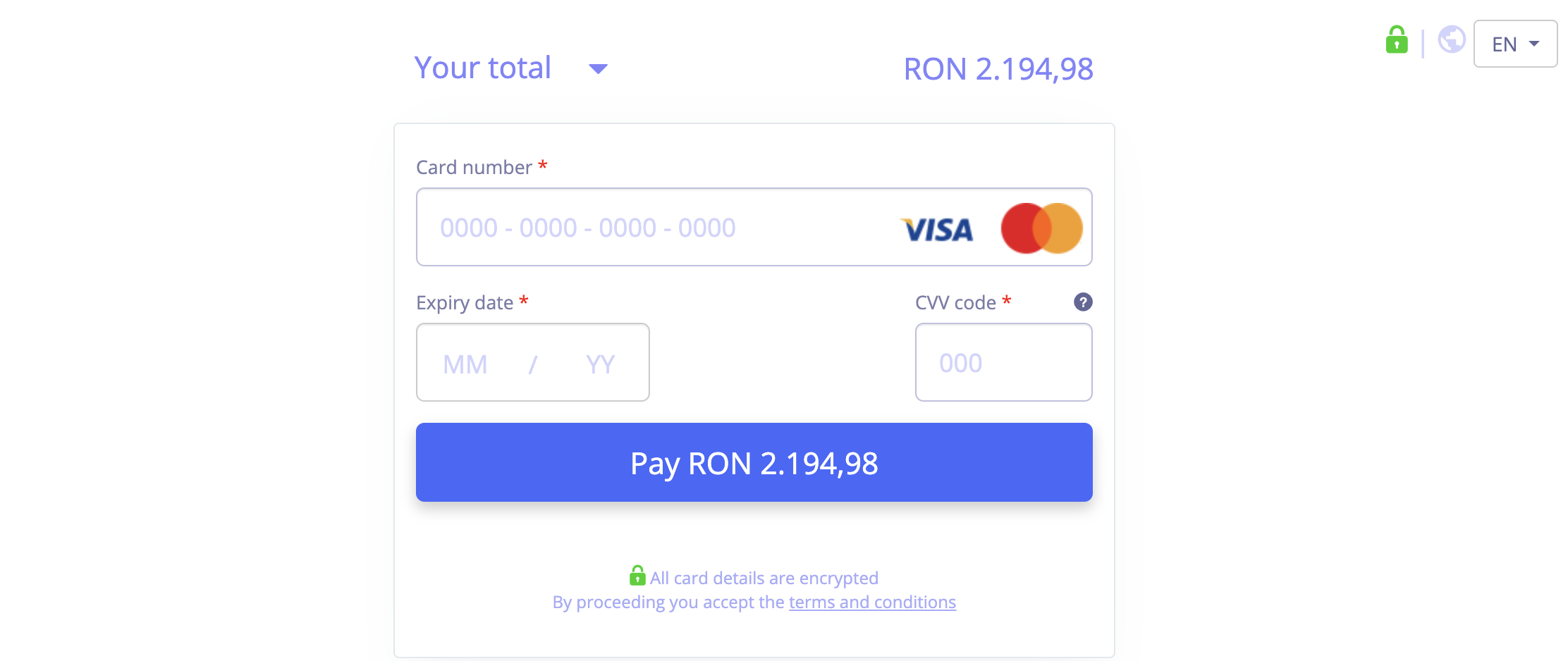
Checkout parameters
Here's a list of all the parameters you can use to configure our hosted checkout page.
Unique identifier of your site profile. Mandatory if more than one site is configured.
The email address to which the invoice will be sent.
Specifies how the card transaction is processed.
auth
: Authorizes the card but does not capture the funds immediately. This is useful for verifying card details and holding funds before completing the purchase. A subsequent capture operation is required to settle the payment.authAndCapture
: Authorizes and immediately captures the funds. This is the standard mode for most transactions where payment is collected at the time of purchase.
The ID of a stored card belonging to the current customer. If provided, the payment will be made using this card.
URL to which the cardholder will be redirected after completing a 3D Secure or digital wallet payment.
Custom data associated with the order. This data will be included in the IPN (Instant Payment Notification) callback, allowing you to pass and retrieve application-specific information related to the order.