Accepting Payments in Your Mobile App
This guide outlines how to integrate xMoney payment processing into your native mobile application using a WebView component. This method allows you to leverage xMoney's secure payment gateway for seamless in-app transactions.
Prerequisites:
- Native Mobile Application: You must have a functioning mobile app (iOS or Android).
- API Key & Site ID:
- Obtain your Private Key and Site ID from your xMoney Dashboard.
- Backend Server: You need a backend server to manage:
- Order, customer, transaction, and card data.
- API endpoints for:
- Generating the JSON request, checksum, and xMoney redirection URL (
backUrl
). - Updating transaction statuses and displaying result messages.
- IPN Listener (Webhook)
- Generating the JSON request, checksum, and xMoney redirection URL (
Payment Flow:
- Customer Adds Items and Proceeds to Checkout:
- The customer adds desired products to their virtual cart within your mobile app.
- They tap the "Checkout" or "Purchase" button to initiate the payment process.
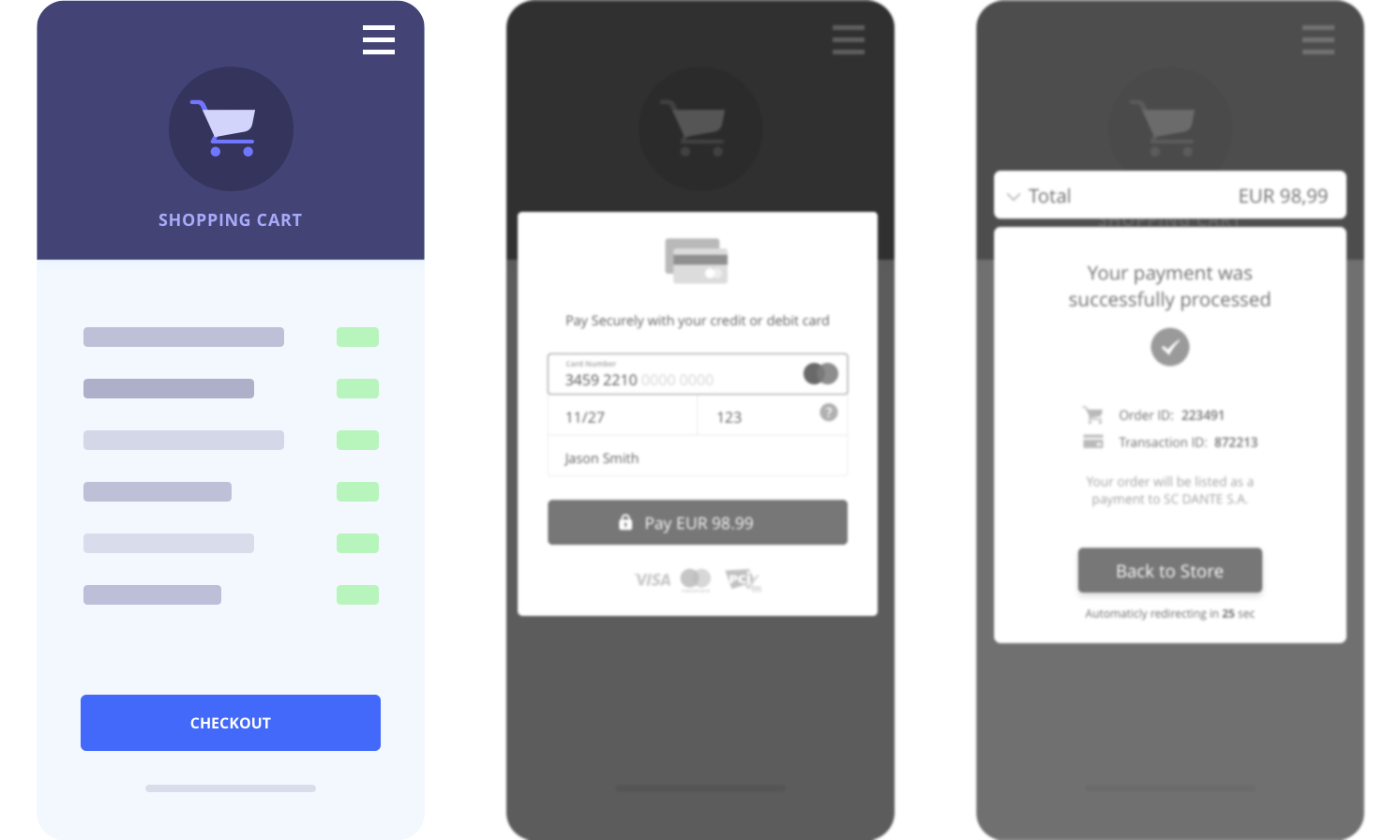
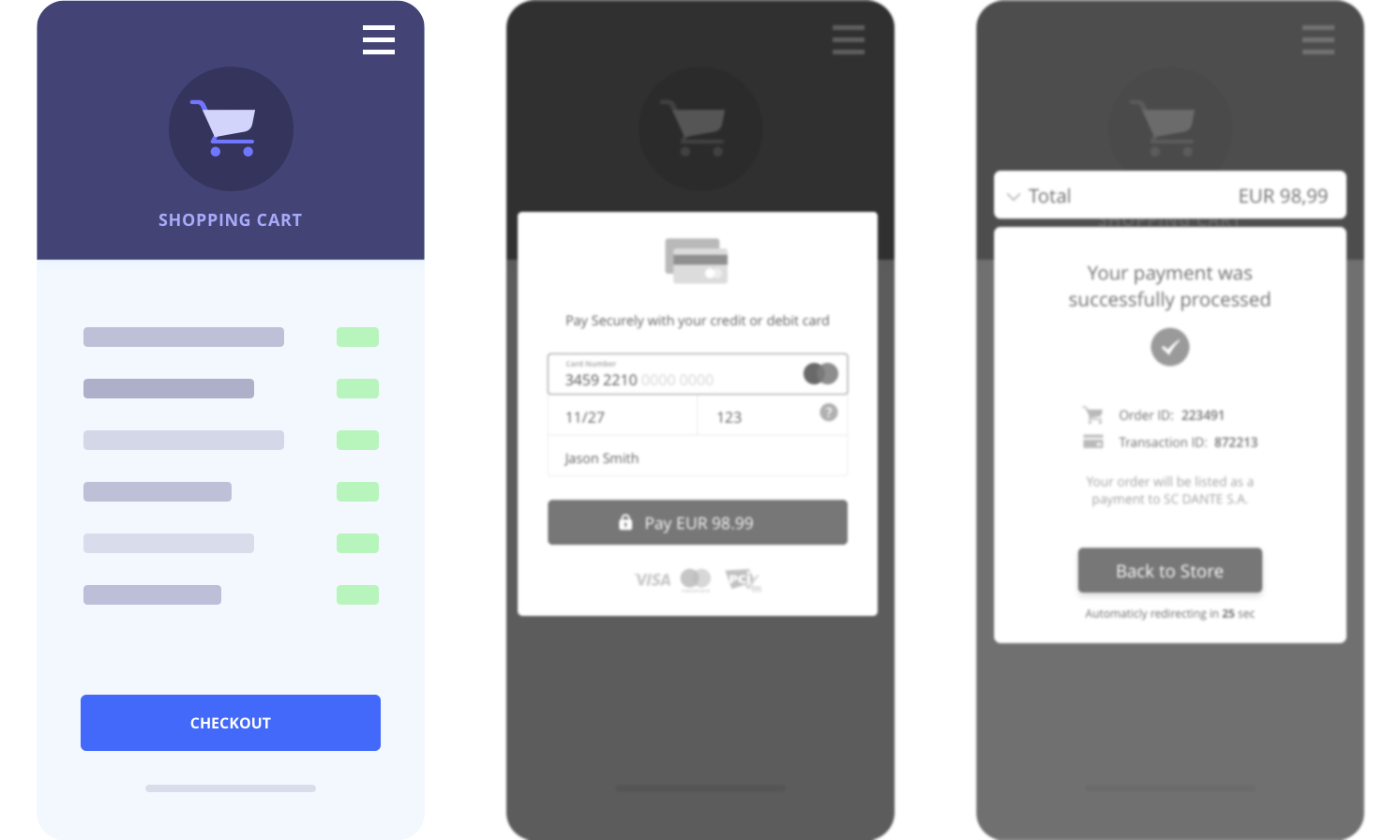
- App Sends Order Request to Your Server:
- The mobile app sends a request to your backend server to create an internal order record.
- This record includes essential details: order number, customer information, cart contents, order amount, etc.
- Important: Assign unique IDs to orders, customers, transactions, and cards (e.g., auto-incrementing numbers or unique strings).
- Set the initial transaction status to "Payment Pending."
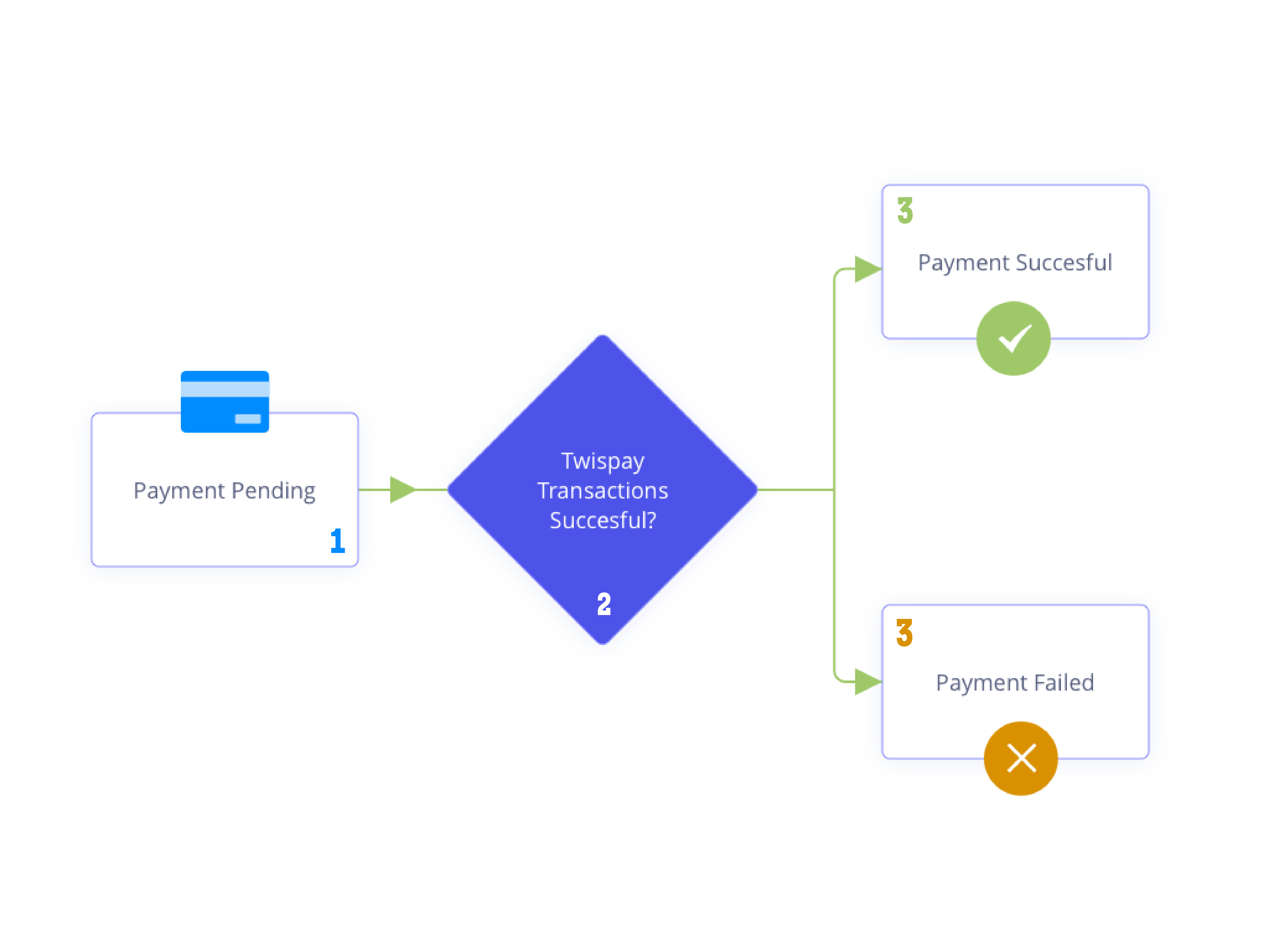
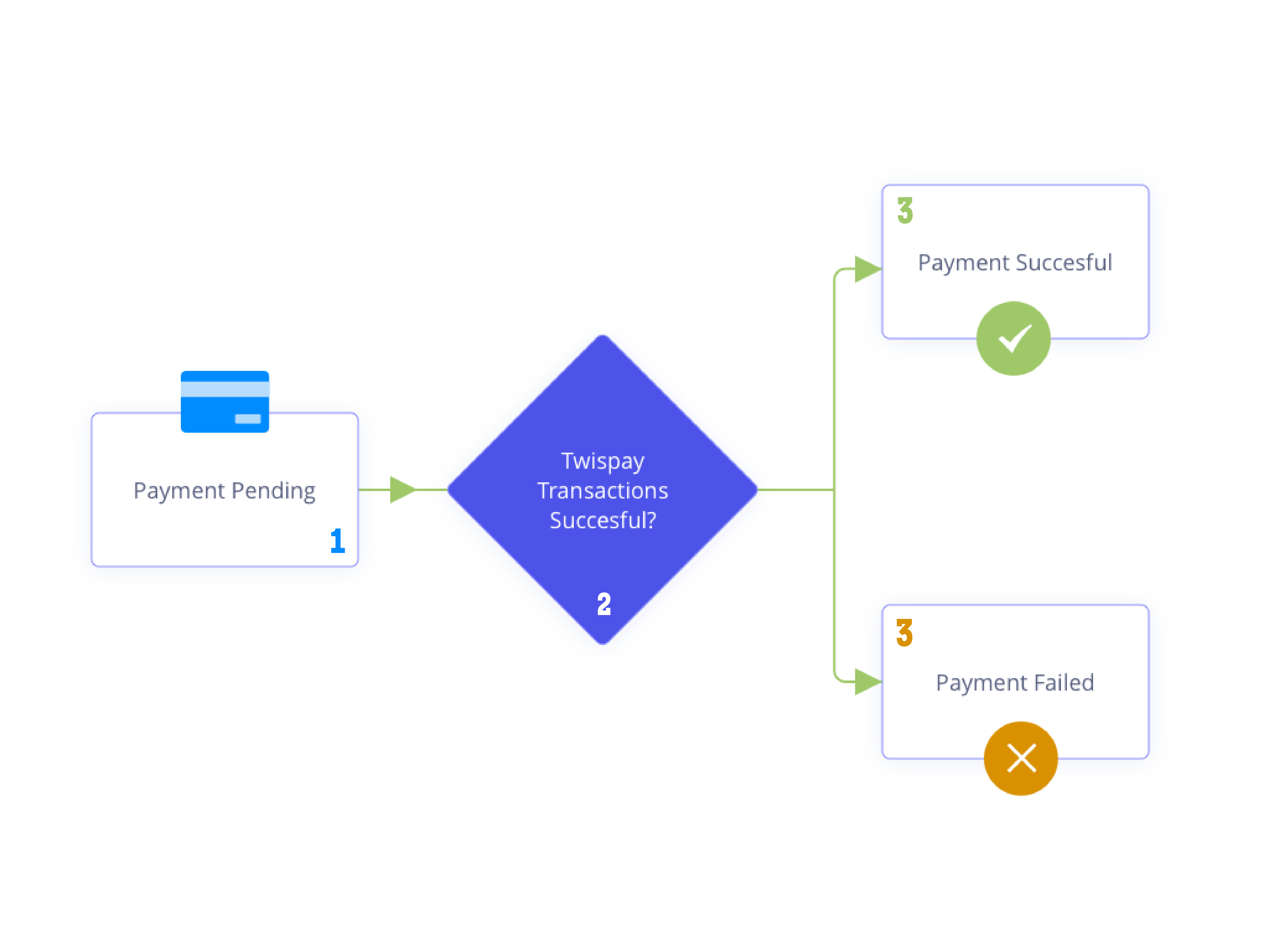
Server Generates xMoney Request Parameters:
Your server generates two crucial parameters:
- JSON Order Data: A JSON object containing all transaction details required by xMoney. Example:
{ "siteId": 1, "customer": { "identifier": "1", "firstName": "John", "lastName": "Doe", "country": "RO", "city": "Bucharest", "phone": "0012120000000", "email": "john.doe@test.com" }, "order": { "orderId": "ID", "description": "Description", "type": "purchase", "amount": 2194.98, "currency": "RON", "description": "order description" }, "cardTransactionMode": "authAndCapture", "backUrl": "[http://your-server.com/payment-result](http://your-server.com/payment-result)" }
- Checksum: An alphanumeric sequence that verifies the JSON's integrity. xMoney uses this to prevent tampering. Example (Java):
String base64JsonRequest = xMoney.getBase64JsonRequest(jsonOrderData); String base64Checksum = xMoney.getBase64Checksum(jsonOrderData, secretKey.getBytes(StandardCharsets.UTF_8));
Server Returns Parameters to Mobile App:
- Your server sends the
base64JsonRequest
,base64Checksum
, and the xMoney secure URL (e.g.,https://secure-stage.xmoney.com
) back to the mobile app.
- Your server sends the
Mobile App Loads Hidden WebView Form:
The mobile app uses a WebView to render a hidden HTML form containing the xMoney parameters. Example:
<form name="form1" action="[https://secure-stage.xmoney.com](https://secure-stage.xmoney.com)" method="post" accept-charset="UTF-8"> <input type="hidden" name="jsonRequest" value="[base64JsonRequest]"> <input type="hidden" name="checksum" value="[base64Checksum]"> <input type="submit" value="Pay"> </form> <script> document.form1.submit(); </script>
The JavaScript
onload
event automatically submits the form, eliminating the need for user interaction.
xMoney Processes Payment:
- xMoney validates the parameters and displays the payment form within the WebView.
- The customer enters their credit card details and submits the form.
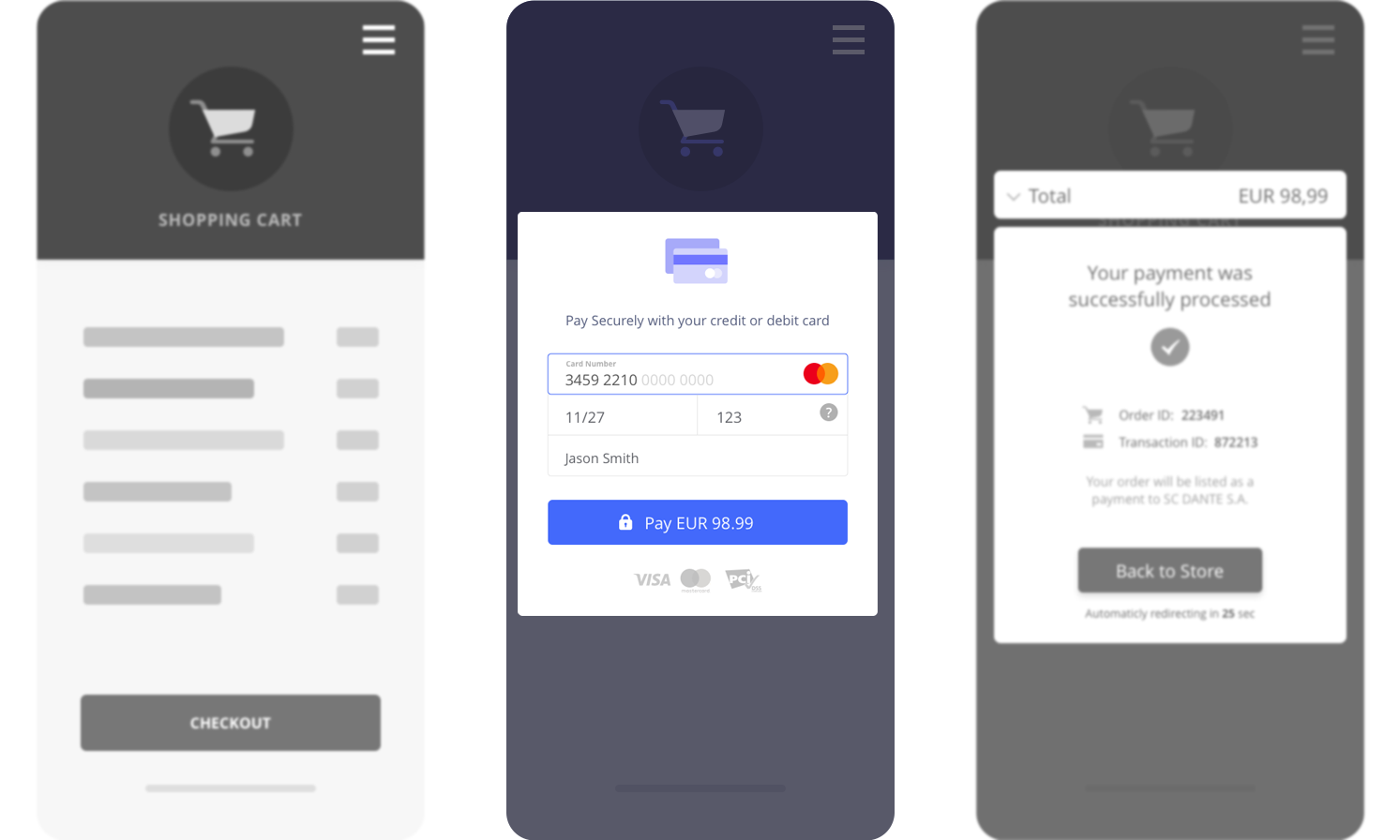
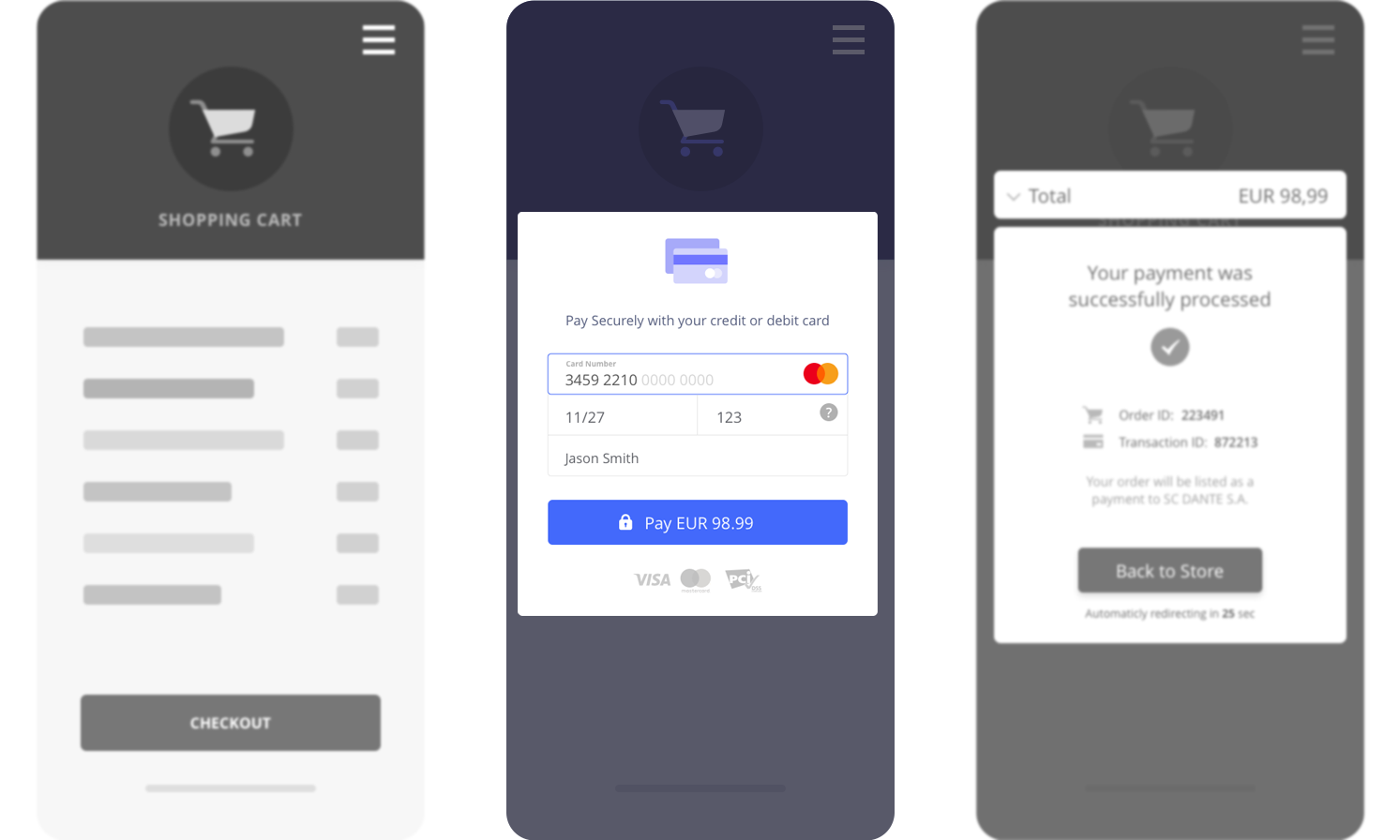
- xMoney Redirects to Your Server's Back URL:
- Upon payment processing, xMoney redirects the WebView to your specified
backUrl
, sending an encryptedopensslResult
parameter via POST. - Important: The
backUrl
must be publicly accessible on your server.
- Upon payment processing, xMoney redirects the WebView to your specified
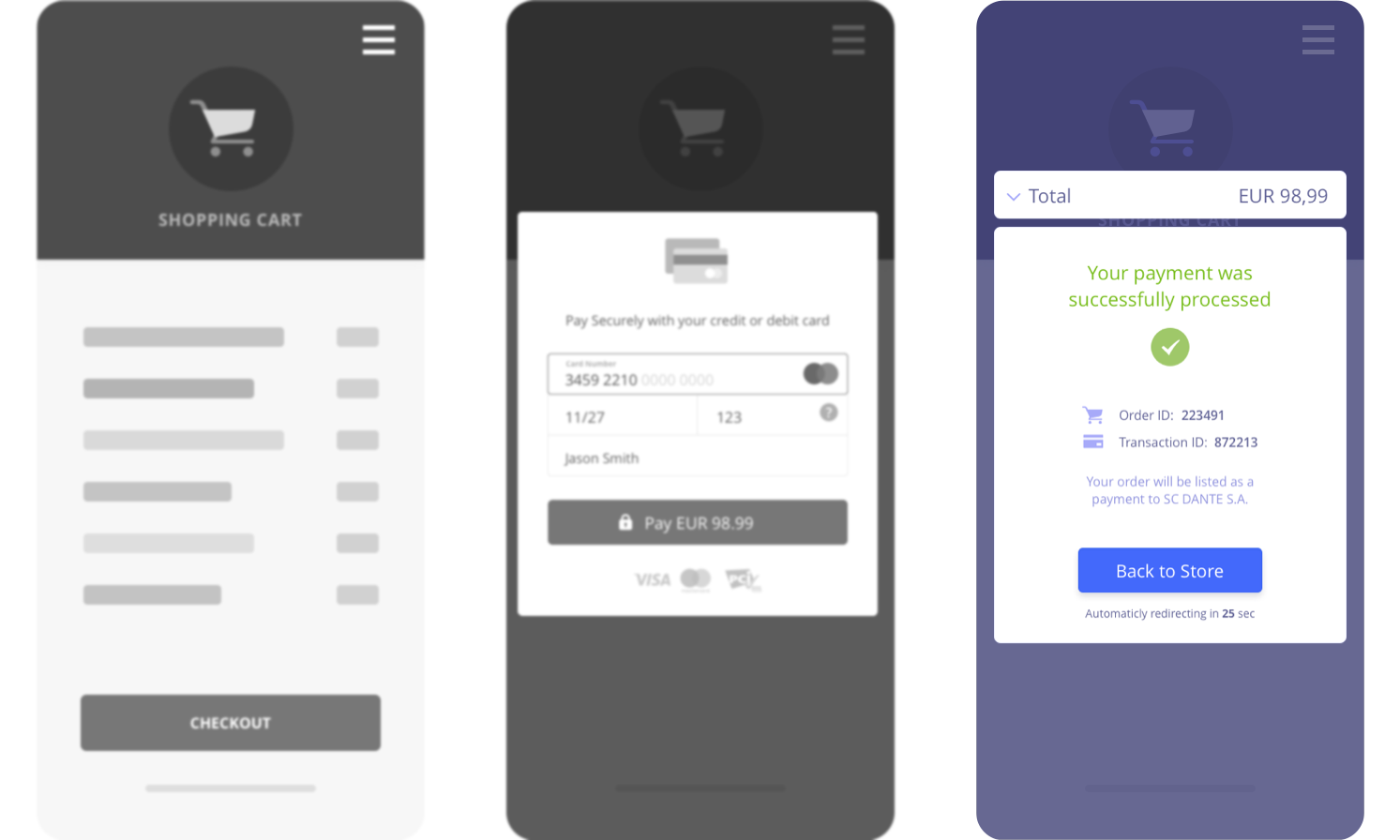
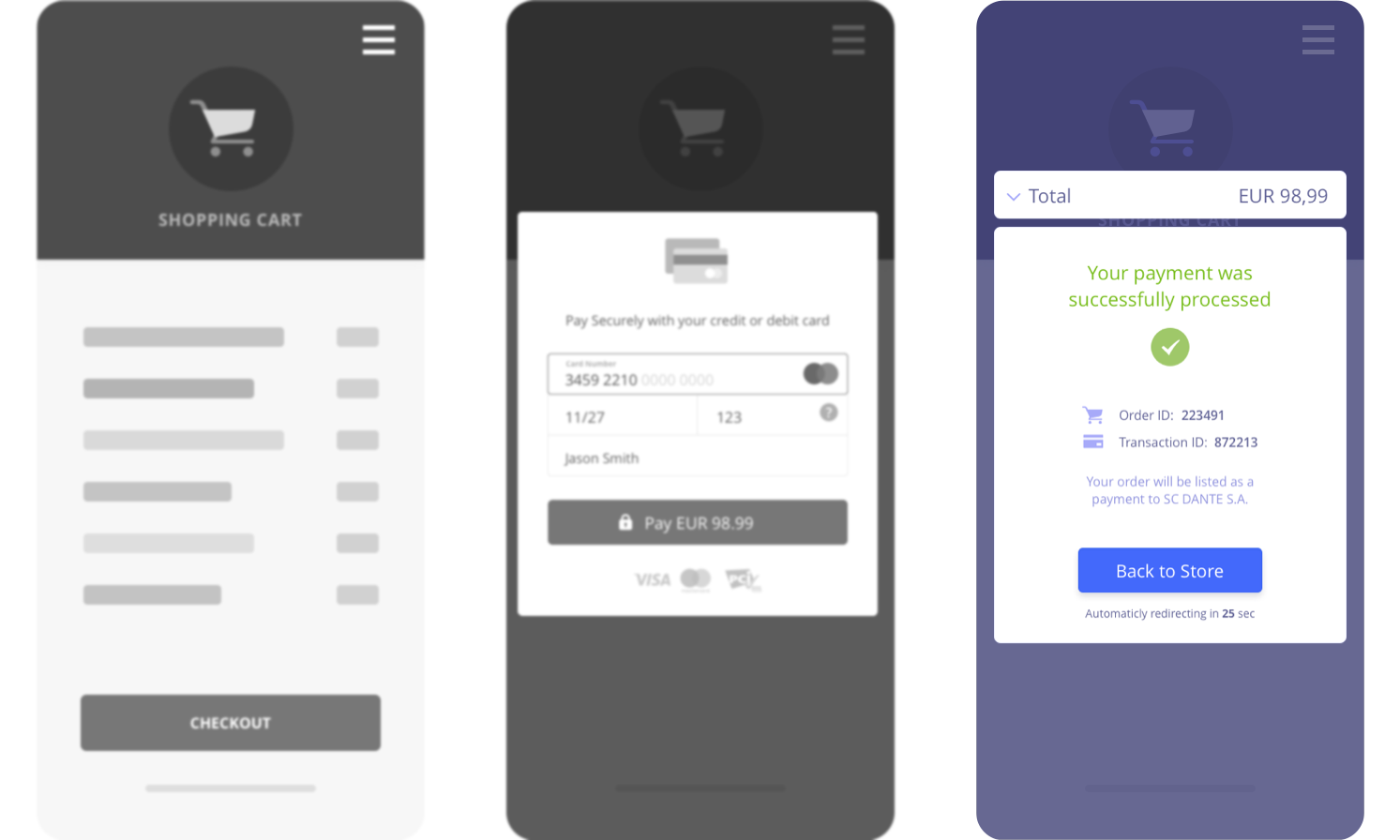
Server Decrypts and Updates Transaction Status:
- Your server decrypts the
opensslResult
to obtain the payment status and other relevant information. - Update the transaction status in your internal system accordingly (e.g., "Payment Successful" or "Payment Failed").
- Display a confirmation or error message to the customer within the WebView.
- Your server decrypts the
Mobile App Closes WebView:
- The mobile app closes the WebView.
Data Reconciliation:
- Store the Customer ID, Card ID, and Transaction ID & Status from xMoney. This creates a double linkage between your system and xMoney's. This allows for data reconciliation in case of sync issues.
Returning Customers (One-Click Payments):
- xMoney supports one-click payments for returning customers who have saved their card details.
- Your server can send a POST order request to the xMoney API with the customer's Card ID and Customer ID.
- Refer to Tokenized payments for details on one-click payments.